Clearing Cloudflare cache using PowerShell in Azure DevOps Pipelines
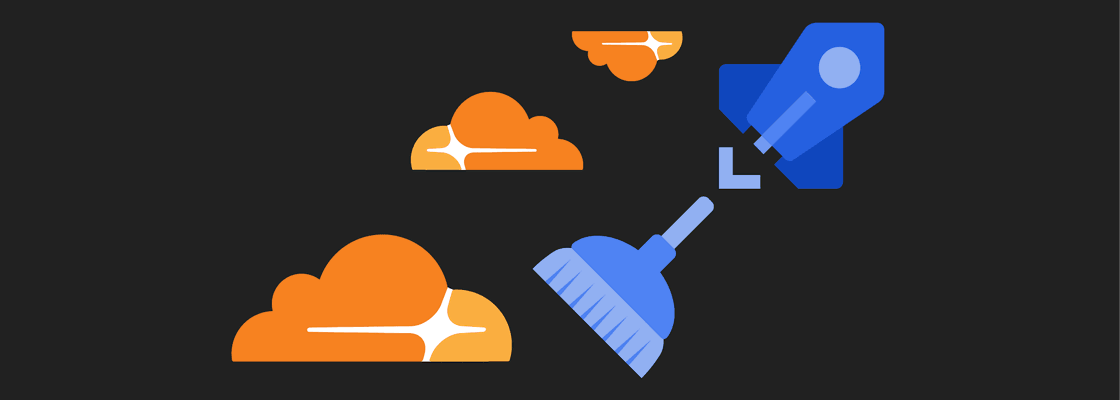
The following post assumes you already have your Cloudflare and Azure Pipelines setup.
When I was deploying changes to an application, the changes didn't appear immediately to the customers. This could've been due to many reasons, but usually browser caching. In this case the changes still didn't show up after clearing the browser cache.
So what was caching the old version of the site? The web server, the database, the load balancer, or the proxy server?
This is a common scenario in the life of a developer or sysadmin. It turns out I deployed the changes without following the lengthy deployment guide which included steps to clear all the layers of caching. Regretting my poor decisions, I decided to automate the process so I can focus on writing code instead of troubleshooting deployments.
One part of the process was to purge Cloudflare's cache, so let's learn how I created a task to clear Cloudflare's caching as part of my Continuous Deployment Azure Pipeline (f.k.a. Visual Studio Team Service, Team Foundation Server).
Creating Clear Cloudflare Cache Task #
Before creating our Clear Cloudflare Cache task, we need to get three variables from Cloudflare to interact with their API.
- Cloudflare Admin Email Address
- Cloudflare API Key
- Cloudflare Zone ID
To get these variables, follow the instructions under "Cloudflare API keys" section from a prior post "Clearing Cloudflare Cache with Cloudflare's API".
In our task we'll use all three variables, which we need to define inside of our pipeline like this:
Now that our variables are stored in our Azure DevOps Pipeline, we can continue on to creating our task.
Navigate to our pipeline, and add a PowerShell task like this:
Let's configure the task as below:
Display name: Bust Cloudflare Cache
Type: Inline
Script: For our script we'll use a modified version of the PowerShell script from a prior post.
The modified version wraps the logic in a function and passes on environment variables that we'll setup after.
Function BustCloudflareCache{ Param( [parameter(Mandatory=$true)] [string] $AdminEmail, [parameter(Mandatory=$true)] [string] $ApiKey, [parameter(Mandatory=$true)] [string] $ZoneId ); $PurgeCacheUri = "https://api.cloudflare.com/client/v4/zones/$ZoneId/purge_cache"; $RequestHeader = @{ 'X-Auth-Email' = $AdminEmail 'X-Auth-Key' = $ApiKey }; $RequestBody = '{"purge_everything":true}'; Invoke-RestMethod ` -Uri $PurgeCacheUri ` -Method Delete ` -ContentType "application/json" ` -Headers $requestHeader ` -Body $RequestBody } BustCloudflareCache -AdminEmail $Env:CloudflareAdminEmail -ApiKey $Env:CloudflareApiKey -ZoneId $Env:CloudflareZoneId
The PowerShell function does the following things:
- Construct the API URL to the cache_purge action, embedding your Zone ID within
- Setting up the required authentication headers which specifies your admin email and the API key
- Setting up the HTTP body to a JSON string specifying to purge everything
- Putting it all together and send an HTTP DELETE request
For more details, read the Cloudflare API documentation for cache_purge.
Environment Variables:
The PowerShell script passes three environment variables to the function, but at the moment those variables are $Null.
To define the environment variables we have to pipe the Pipeline variables to the Environment Variables of the PowerShell task.
We can do this by naming our environment variable and then grabbing the Pipeline Variable using the $() syntax.
At execution time $(CloudflareAdminEmail) will be replaced with the value of the Pipeline Variable.
Name | Value |
---|---|
CloudflareAdminEmail | $(CloudflareAdminEmail) |
CloudflareApiKey | $(CloudflareApiKey) |
CloudflareZoneId | $(CloudflareZoneId) |
Now that the task has been setup, we can test it by running the pipeline and the task will purge Cloudflare's cache.
Conclusion #
Using Azure Pipelines we setup a PowerShell task that interacts with Cloudflare's API to clear cache on Cloudflare's edge servers. By including this as part of our deployment pipeline, we have less manual steps to perform or forget about.
After purging the cache, we could now add another PowerShell task that crawls the website to re-hydrate Cloudflare's cache (ex1, ex2).
Happy automating!