PowerShell Snippet: Clearing Cloudflare Cache with Cloudflare's API
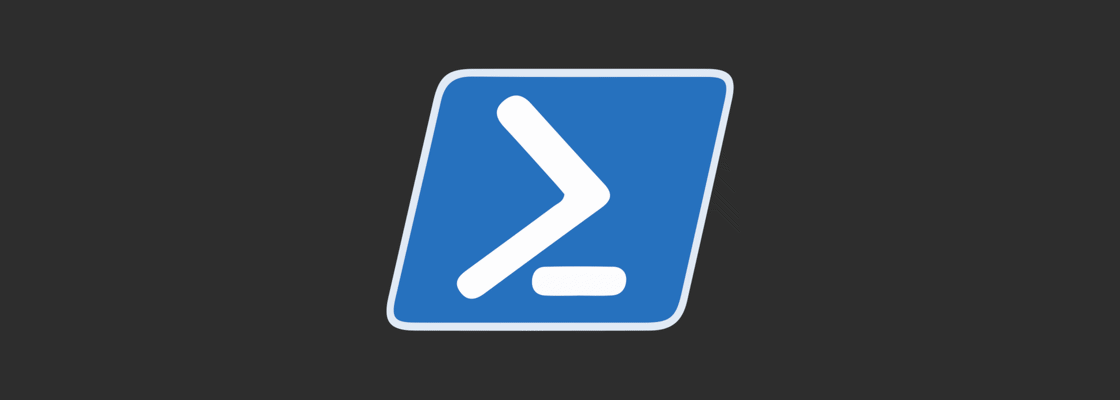
Cloudflare has many services to help speed up your web properties, scale globally, secure your site, and much more. One way Cloudflare improves the speed of your website is by caching your website closer to visitors. By default HTML isn't being cached, but using Cloudflare PageRules you can also get HTML cached. Whenever you cache something, there comes a time you'll need to refresh, purge, or clear that cache. Cloudflare provides a GUI to do this, but every action you can perform using the GUI, you can also do with Cloudflare's API. You could use the API to auto purge the cache whenever you update content in your CMS of choice, or purge the cache as part of your Continuous Delivery pipeline. Using PowerShell we'll interact with Cloudflare's API and purge their cache.
Cloudflare API keys
Before being able to interact with the Cloudflare API, you'll need to acquire the necessary keys. For the scripts you'll need to acquire the Zone ID, and the Global API Key.
Navigate to the overview page of your site in Cloudflare and scroll down to find the Zone ID at the bottom right.
After copying your Zone ID, click on 'Get your API key' which will take you to your profile. At the bottom of your profile, you'll find the following section:
Click on 'View' for the Global API key and follow the instructions to obtain the key. Keep in mind with this API key, you can perform all Cloudflare actions, for all your Cloudflare sites. Cloudflare is making API keys to be more scoped to specific permissions and sites, but for now keep this risk in mind!
With the API key and Zone ID in your possession, you can move on to interacting with the API.
Purging Cloudflare cache using API #
Using the script below you can clear all the Cloudflare cache for your website:
Param( [parameter(Mandatory=$true)] [string] $AdminEmail, [parameter(Mandatory=$true)] [string] $ApiKey, [parameter(Mandatory=$true)] [string] $ZoneId ); $PurgeCacheUri = "https://api.cloudflare.com/client/v4/zones/$ZoneId/purge_cache"; $RequestHeader = @{ 'X-Auth-Email' = $AdminEmail 'X-Auth-Key' = $ApiKey }; $RequestBody = '{"purge_everything":true}'; Invoke-RestMethod ` -Uri $PurgeCacheUri ` -Method Delete ` -ContentType "application/json" ` -Headers $requestHeader ` -Body $RequestBody
The PowerShell script above does the following:
- Construct the API URL to the cache_purge action, embedding your Zone ID within
- Setting up the required authentication headers which specifies your admin email and the API key
- Setting up the HTTP body to a JSON string specifying to purge everything
- Putting it all together and send an HTTP DELETE request
For more details, read the Cloudflare API documentation for cache_purge.
Using the following script you clear cache for specific URLs:
Param( [parameter(Mandatory=$true)] [string[]] $UrlsToPurge, [parameter(Mandatory=$true)] [string] $AdminEmail, [parameter(Mandatory=$true)] [string] $ApiKey, [parameter(Mandatory=$true)] [string] $ZoneId ); $PurgeCacheUri = "https://api.cloudflare.com/client/v4/zones/$ZoneId/purge_cache"; $RequestHeader = @{ 'X-Auth-Email' = $AdminEmail 'X-Auth-Key' = $ApiKey }; $RequestBody = @{ files = $UrlsToPurge } | ConvertTo-Json -Compress; Invoke-RestMethod ` -Uri $PurgeCacheUri ` -Method Delete ` -ContentType "application/json" ` -Headers $requestHeader ` -Body $RequestBody
This function pretty much does the same as the previous snippet, except that it will construct a different JSON payload that includes all the URLs passed on to it.
For more details, read the Cloudflare API documentation for cache_purge by URLs.