How to deploy Blazor WebAssembly to DigitalOcean App Platform
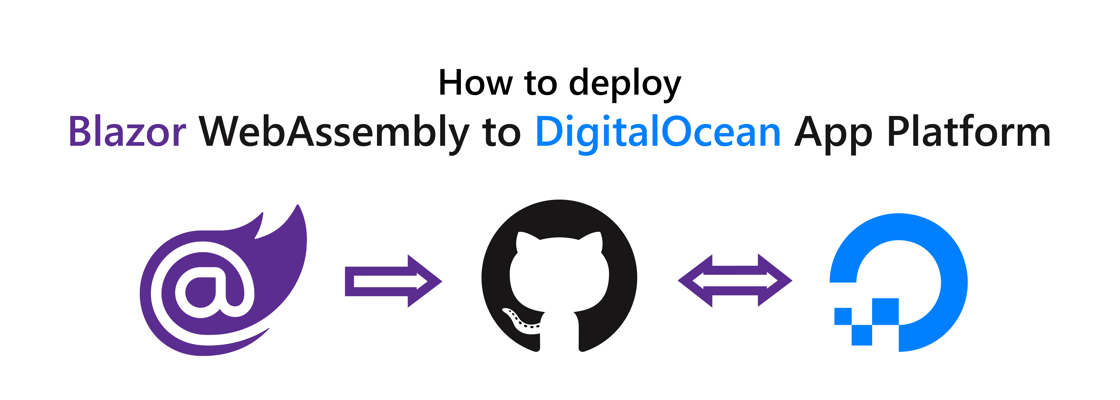
With ASP.NET Blazor WebAssembly (WASM) you can create .NET web applications that run completely inside of the browser sandbox. The published output of a Blazor WASM project are static files. Now that you can run .NET web applications without server-side code, you can deploy these applications to various static site hosts, such as DigitalOcean App Platform.
Here are some other tutorials if you're interested in hosting Blazor WASM on other static site hosts:
- Azure Static Web Apps using GitHub
- Azure Static Web Apps using Azure DevOps (by Dave Brock)
- AWS Amplify
- Netlify
- Cloudflare Pages
- Heroku
- GitHub Pages
- Firebase Hosting
This walkthrough will show you how to deploy Blazor WASM to DigitalOcean App Platform.
App Platform is a Platform-as-a-Service (PaaS) offering that allows developers to publish code directly to DigitalOcean servers without worrying about the underlying infrastructure.
Source: DigitalOcean
You can host multiple workloads using App Platform, such as services (workloads publicly accessible via HTTP), workers (workloads not publicly accessible via HTTP), and Static Sites for hosting static web content.
The App Platform can pull down your code from multiple Git providers and docker registries, then build your code on their build platform, and upload the resulting static files to their static site service.
This guide will walk you through these high-level steps:
- Create Blazor WebAssembly project
- Commit the project to a Git repository
- Create a new GitHub project and push the Git repository to GitHub
- Create a new DigitalOcean App using the DigitalOcean Command Line Interface
- Build Blazor WASM using Docker
Prerequisites:
- .NET CLI
- Git
- GitHub account
- DigitalOcean account
- DigitalOcean command-line interface (doctl) (installation instructions)
You can find the source code for this guide on GitHub.
Create Blazor WebAssembly project
Run the following commands to create a new Blazor WASM project:
mkdir BlazorWasmDigitalOcean cd BlazorWasmDigitalOcean dotnet new blazorwasm
To give your application a try, execute dotnet run
and browse to the URL in the output (probably https://localhost:5001):
dotnet run # Building... # info: Microsoft.Hosting.Lifetime[0] # Now listening on: https://localhost:5001 # info: Microsoft.Hosting.Lifetime[0] # Now listening on: http://localhost:5000 # info: Microsoft.Hosting.Lifetime[0] # Application started. Press Ctrl+C to shut down. # info: Microsoft.Hosting.Lifetime[0] # Hosting environment: Development # info: Microsoft.Hosting.Lifetime[0] # Content root path: C:\Users\niels\source\repos\BlazorWasmDigitalOcean # info: Microsoft.Hosting.Lifetime[0] # Application is shutting down...
Optional: You can use the dotnet publish
command to publish the project and verify the output:
dotnet publish # Microsoft (R) Build Engine version 16.8.0+126527ff1 for .NET # Copyright (C) Microsoft Corporation. All rights reserved. # # Determining projects to restore... # All projects are up-to-date for restore. # BlazorWasmDigitalOcean -> C:\Users\niels\source\repos\BlazorWasmDigitalOcean\bin\Debug\net5.0\BlazorWasmDigitalOcean.dll # BlazorWasmDigitalOcean (Blazor output) -> C:\Users\niels\source\repos\BlazorWasmDigitalOcean\bin\Debug\net5.0\wwwroot Optimizing assemblies for size, which may change the behavior of the app. Be sure to test after publishing. See: https://aka.ms/dotnet-illink # Compressing Blazor WebAssembly publish artifacts. This may take a while... # BlazorWasmDigitalOcean -> C:\Users\niels\source\repos\BlazorWasmDigitalOcean\bin\Debug\net5.0\publish\
In the publish directory, you will find a web.config file and a wwwroot folder. The config file helps you host your application in IIS, but you don't need that file for static site hosts. Everything you need will be inside of the wwwroot folder. The wwwroot folder contains the index.html, CSS, JS, and DLL files necessary to run the Blazor application.
Push Blazor project to GitHub
For this walkthrough, your application source code must be inside of a GitHub repository.
First, you need to create a local Git repository and commit your source code to the repository using these commands:
# add the gitignore file tailored for dotnet applications, this will ignore bin/obj and many other non-source code files dotnet new gitignore # create the git repository git init # track all files that are not ignore by .gitignore git add --all # commit all changes to the repository git commit -m "Initial commit"
Create a new GitHub repository (instructions) and copy the commands to "push an existing repository from the command line" from the empty GitHub repository page, here's what it should look like but with a different URL:
git remote add origin https://github.com/Swimburger/BlazorWasmDigitalOcean.git git push -u origin main
Add Dockerfile to build Blazor WASM #
When you create a static site using the App Platform, you can provide a build command which will be used to build your static site before deploying it to the static site host.
Unfortunately, the build command option does not work for .NET applications. Before even running the build command, the App Platform gives up and complains about not finding an app from their list of supported languages and frameworks.
.NET isn't one of the supported platforms for App Platform. Luckily, there's an alternative way to build and run .NET apps on the App Platform: Docker containers
Instead of providing a build command, you can provide a dockerfile to build your Blazor WASM application. This feature isn't accessible using the GUI, but you can use the doctl to create the app and specify the dockerfile that way.
Before doing that, let's create the dockerfile. Create a new file called 'Dockerfile.build' in your project with the following content:
FROM mcr.microsoft.com/dotnet/sdk:5.0 WORKDIR /app COPY BlazorWasmDigitalOcean.csproj BlazorWasmDigitalOcean.csproj RUN dotnet restore BlazorWasmDigitalOcean.csproj COPY . . RUN dotnet publish -c Release -o /output --no-restore --nologo
Feel free to learn more about docker and .NET at Microsoft's documentation, but you can keep going without knowing docker or having docker installed locally.
Essentially, this docker file does the following:
- A container is created from the .NET 5 SDK image provided by Microsoft. All subsequent instructions will be run inside a container containing the .NET 5 SDK.
- Change the current working directory in the container to '/app'.
- Copy the csproj-file to the container so you can restore the packages listed in the file
- Run the
dotnet restore
command to restore all packages - Copy all files from the current folder '.' outside of the container to the working directory inside the container. This will copy all your source code to the '/app' folder inside the container.
- Run the
dotnet publish
command with the following arguments:- -c: Use the 'Release' configuration which will optimize the Blazor project.
- -o: Put the publish output in the '/output' folder
- --no-restore: Skip the restore step since the packages have been restored earlier.
- --nologo: Removes some unneeded output
You can restore, build, and publish all in a single command, but to optimize for Docker's caching features, the restore has been separated from the publish command.
The App Platform will look for the dockerfile in your source code, so you need to commit the file to Git and push the change to GitHub:
git add --all git commit -m "Add dockerfile.build" git push
Next up, you'll create a static site app using doctl and App Platform YAML configuration.
Create the DigitalOcean App #
Make sure you have installed the DigitalOcean command-line interface (doctl) (installation instructions) and authenticate using the doctl auth init
command if you haven't already.
Create a YAML file at '.do/app.yaml' with the following content:
name: swimburger-blazor-wasm static_sites: - name: site github: repo: Swimburger/BlazorWasmDigitalOcean branch: main deploy_on_push: true dockerfile_path: Dockerfile.build output_dir: /output/wwwroot index_document: index.html catchall_document: index.html
Update the properties in the YAML file:
- name: Enter a name that is unique across your account. Use your username suffixed with '-blazor-wasm'.
- static_sites
- name: Enter a name that is unique across the different components in this file. You can have multiple "components" such as workers, services, static_sites, etc.
When you update the YAML file in the future, this name will be used to make the changes to the correct component. Since there's only one component, any name will do. - github
- repo: enter your [GITHUB_USERNAME]/[GITHUB_REPOSITORY]
- branch: specify the name of your main branch
- deploy_on_push: When set to 'true', every time push to the branch specified above, the App Platform will rebuild and deploy your application
- dockerfile_path: The path relative from the root of Git repository pointing to the location of your dockerfile. Your dockerfile should be located at 'Dockerfile.build'.
- output_dir: Specify the location of your static site after the build command or dockerfile is run. In 'Dockerfile.build' the Blazor WASM application is published to '/output', but the actual Blazor App and its static files are in a subfolder called 'wwwroot'.
Use '/output/wwwroot' for this property. - index_document: Use 'index.html' for this property.
- catchall_document: Also use 'index.html' for this property. When no HTML file is found at the requested path, the catch_all document will be returned which is exactly what you need for Blazor WASM.
- name: Enter a name that is unique across the different components in this file. You can have multiple "components" such as workers, services, static_sites, etc.
Run the following command to create the application on App Platform:
doctl app create --spec .do/app.yaml
Navigate to the Apps section in DigitalOcean and click on your new application.
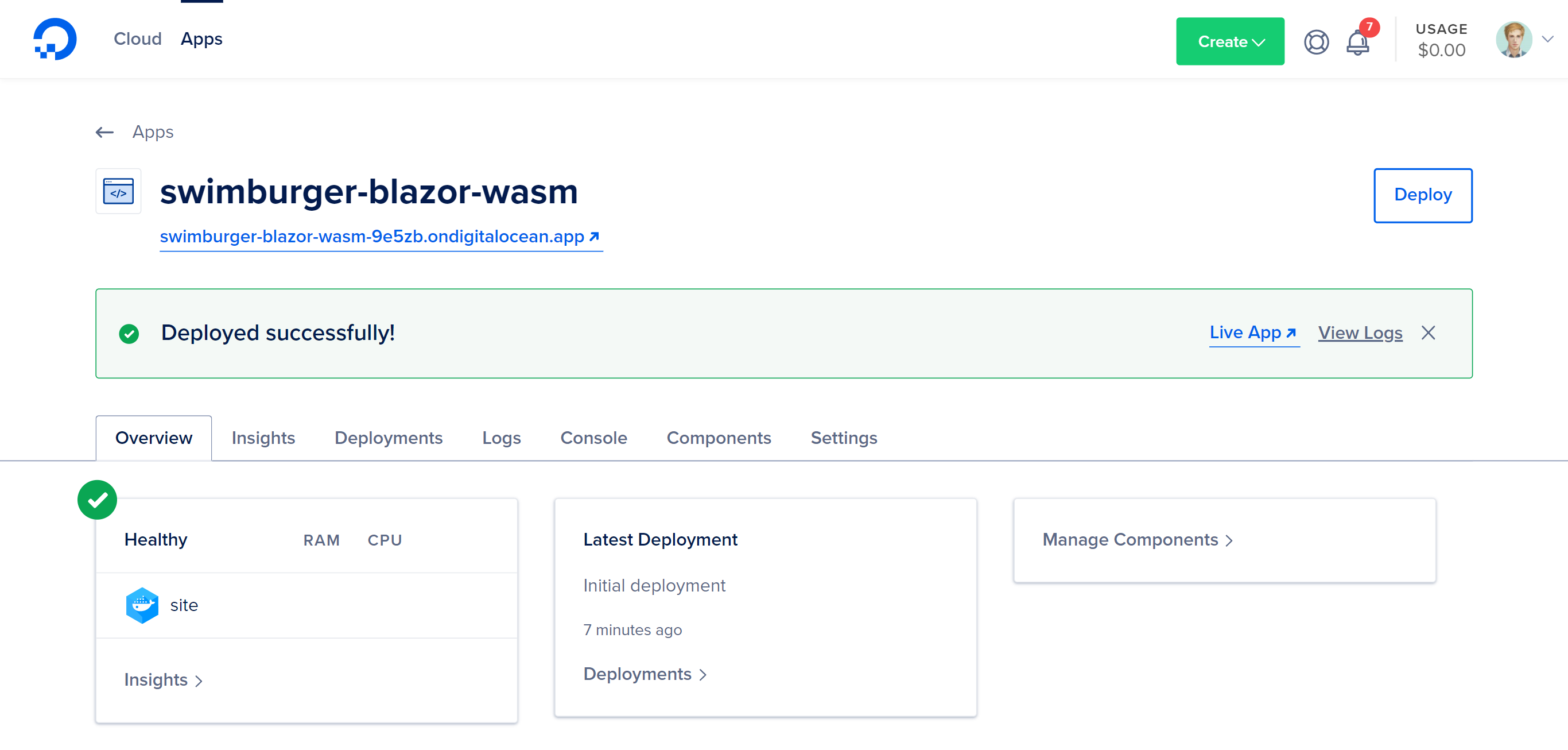
You can watch the deployment logs stream in real-time while you wait for your application to be deployed. When the deployment is finished, click on "Live App" to verify your Blazor WASM application is running.
Summary #
Blazor WebAssembly can be served as static files. These files can be hosted in static hosting services such as DigitalOcean App Platform. You can create a new DigitalOcean App Platform static site using GitHub as the source code provider. DigitalOcean's App Platform does not contain .NET support so you have to use docker to build your Blazor WASM application. The App Platform will upload the static files of the Blazor WASM application in the docker container to the static site.