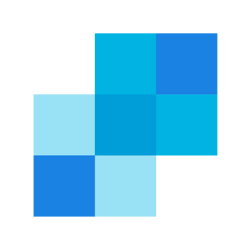
How to send recurring emails in C# .NET using SendGrid and Quartz.NET
-
.NET
Learn how to send recurring emails with C# .NET using Quartz.NET and SendGrid's APIs
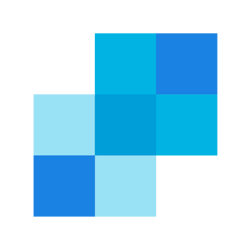
Send Emails using the SendGrid API with .NET 6 and C#
-
.NET
Learn how to send emails using the SendGrid API with a .NET 6 console application and C#
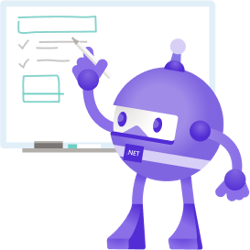
Generic Linear Search/Sequential Search for a sequence in C# .NET
-
.NET
To practice algorithms and data structures, I reimplemented Linear Search/Sequential Search for a sequence using C#'s generic type parameters.
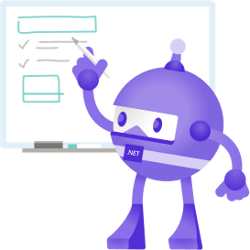
Generic Boyer–Moore–Horspool algorithm in C# .NET
-
.NET
To practice algorithms and data structures, I reimplemented Boyer–Moore–Horspool algorithm for a sequence using C#'s generic type parameters.
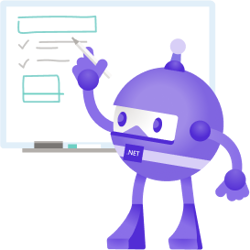
Generic Binary Search in C# .NET
-
.NET
To practice algorithms and data structures, I reimplemented binary search using C#'s generic type parameters.
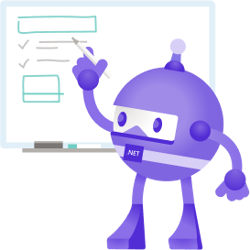
Generic Insertion Sort in C# .NET
-
.NET
To practice algorithms and data structures, I reimplemented insertion sort using C#'s generic type parameters.
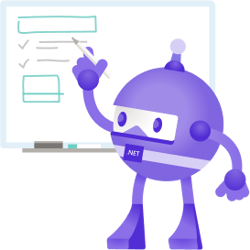
Generic Quick Sort in C# .NET
-
.NET
To practice algorithms and data structures, I reimplemented Quick Sort using C#'s generic type parameters.
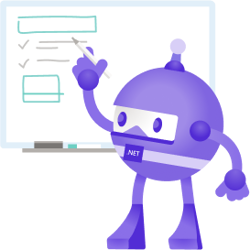
Generic Merge Sort in C# .NET
-
.NET
To practice algorithms and data structures, I reimplemented merge sort using C#'s generic type parameters.
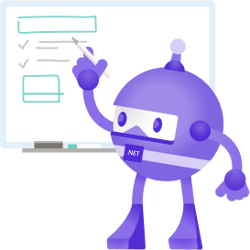
Generic Bubble Sort in C# .NET
-
.NET
To practice algorithms and data structures, I reimplemented bubble sort using C#'s generic type parameters.
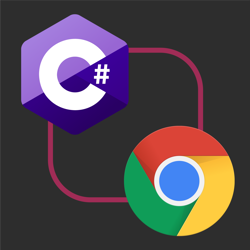
Download the right ChromeDriver version & keep it up to date on Windows/Linux/macOS using C# .NET
-
.NET
Chrome frequently updates automatically causing ChromeDriver versions to mismatch. Using C# .NET you can download the correct version of the ChromeDriver and keep it up-to-date.
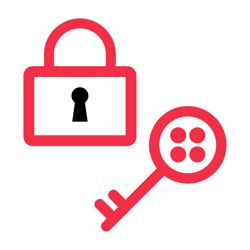
Better Authentication with Twilio API Keys
-
.NET
API Keys are now the preferred way to authenticate with Twilio's API. You can create as many API Keys as you need and remove them if they are compromised or no longer used.
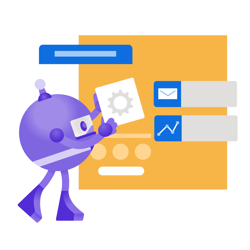
Use project Tye to host Blazor WASM and ASP.NET Web API on a single origin to avoid CORS
-
.NET
Using Microsoft's experimental Project Tye, you configured the proxy to forward requests to '/api' to the Web API, and all other requests to the Blazor WASM client.
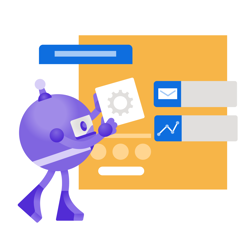
Use YARP to host client and API server on a single origin to avoid CORS
-
.NET
Using Microsoft's new reverse proxy "YARP", you configured the proxy to forward requests to '/api' to the Web API, and all other requests to the Blazor WASM client.
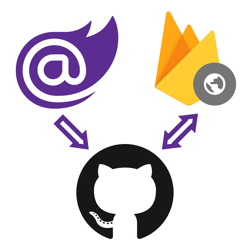
How to deploy Blazor WebAssembly to Firebase Hosting
-
.NET
With ASP.NET Blazor WebAssembly you can create .NET applications that run completely inside of the browser. The output of a Blazor WASM project are all static files. You can deploy these applications to various static site hosts like Firebase Hosting.
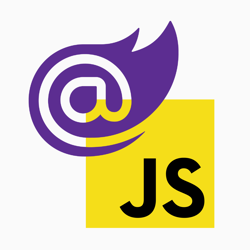
Interacting with JavaScript Objects using the new IJSObjectReference in Blazor
-
.NET
A new type is introduced in .NET 5 called IJSObjectReference. This type holds a reference to a JavaScript object and can be used to invoke functions available on that JavaScript object.
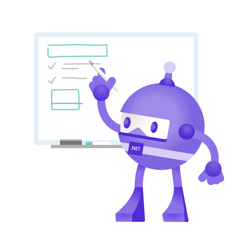
Harden Anti-Forgery Tokens with IAntiforgeryAdditionalDataProvider in ASP.NET Core
-
.NET
Using IAntiforgeryAdditionalDataProvider you can harden ASP.NET Core's anti-forgery token feature by adding additional data and validating the additional data.
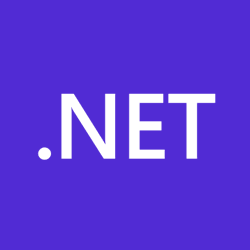
Rethrowing your exceptions wrong in .NET could erase your stacktrace
-
.NET
You may be erasing your stacktrace if you are catching and rethrowing exceptions the wrong way. This could make debugging a nightmare because you don't know where the exception was originally thrown.
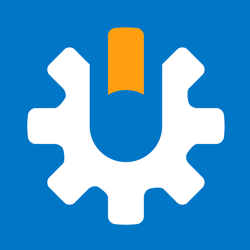
Checking out NDepend: a static code analysis tool for .NET
-
.NET
NDepend is a static code analysis tool (SAST) for .NET. NDepend will analyze your code for code smells, best practices, complexity, dead code, naming conventions, and much more.
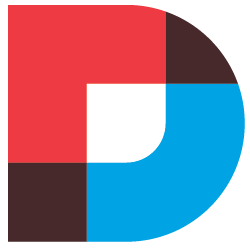
How to add Hangfire to DNN
-
.NET
DNN already has an excellent built-in scheduler you can use to schedule tasks. But you may be more familiar with and prefer Hangfire for running background jobs. These instruction will walk you through configuring Hangfire in with DNN.
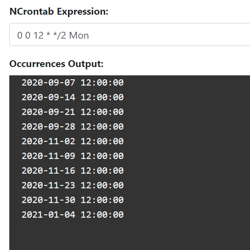
Introducing NCrontab Tester (Blazor WebAssembly)
-
.NET
Introducing NCrontab Expression tester, made using NCrontab .NET library, Blazor WebAssembly, and hosted on Azure Static Web Apps