Bulk add IP Access Restrictions to Azure App Service using Az PowerShell
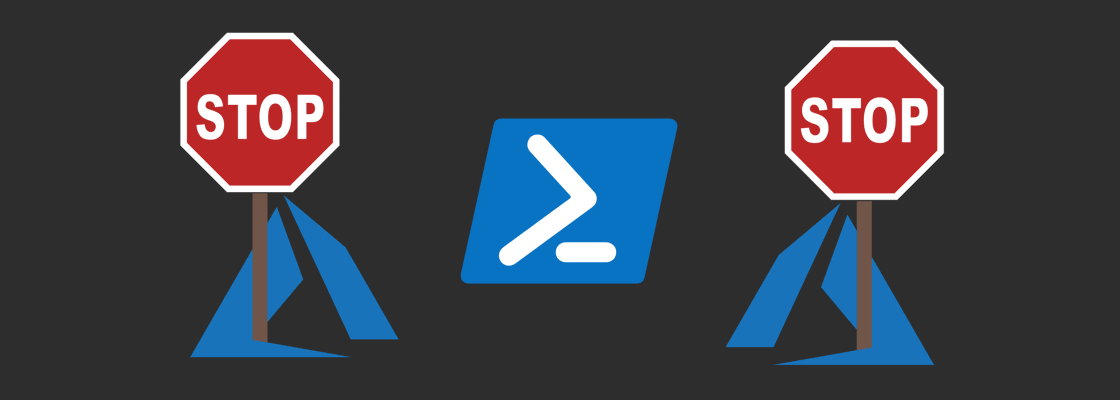
By default Azure App Services are publicly accessible via Azure's public DNS in the format of [APPSERVICE_NAME].azurewebsites.net, but there are many reasons for not wanting your app to be accessible via Azure's default DNS such as:
- To prevent Search Engines from indexing and associating your website content with the wrong domain name
- To enforce all traffic is routed through a Web Application Firewall (WAF)
- Your app service only needs to be accessible to other Cloud resources and leaving it publicly accessible is a security risk
There's a feature called "Access Restrictions" inside of Azure App Service which allows you to tighten down who can communicate with your web application. You can lock your service down to a list of IPv4 ranges, IPv6 ranges, or to your Virtual Network. You can follow Microsoft's documentation to set up these restrictions using the web interface.
Unfortunately, there's no way to bulk insert multiple IP ranges using the GUI, but you can by using PowerShell and the Az PowerShell module.
Bulk insert IP Access Restrictions using Az PowerShell Script #
Currently, there's no built-in function to interact directly with the Access Restrictions in the Az PowerShell module, but with a little PowerShell scripting you can build that functionality. The following script will:
- Log you into Azure
- Fetch your App Service
- Add the new IP Restrictions to the existing restrictions
- Send the updated App Service properties back to Azure Resource Manager (ARM will update the resource for you)
Param( [Parameter(Mandatory = $true)] [string] $ResourceGroupName, [Parameter(Mandatory = $true)] [string] $AppServiceName, [Parameter(Mandatory = $true)] [string] $SubscriptionId, [Parameter(Mandatory = $true)] [Hashtable[]] $NewIpRules #PSObject has parsing bug in az-module, so we ahve to use hashtable instead ) $ErrorActionPreference = "Stop" Import-Module Az #If logged in, there's an azcontext, so we skip login if($Null -eq (Get-AzContext)){ Login-AzAccount } Select-AzSubscription -SubscriptionId $SubscriptionId #grab the latest available api version $APIVersion = ((Get-AzResourceProvider -ProviderNamespace Microsoft.Web).ResourceTypes | Where-Object ResourceTypeName -eq sites).ApiVersions[0] $WebAppConfig = Get-AzResource -ResourceName $AppServiceName -ResourceType Microsoft.Web/sites/config -ResourceGroupName $ResourceGroupName -ApiVersion $APIVersion foreach ($NewIpRule in $NewIpRules) { $WebAppConfig.Properties.ipSecurityRestrictions += $NewIpRule } Set-AzResource -ResourceId $WebAppConfig.ResourceId -Properties $WebAppConfig.Properties -ApiVersion $APIVersion
Usage:
$restrictions = @( @{ ipAddress = "173.245.48.0/20"; action = "Allow"; priority = "100"; name = "some ip"; description = "some ip"; tag = "Default"; }, @{ ipAddress = "2400:cb00::/32"; action = "Allow"; priority = "100"; name = "some ip"; description = "some ip"; tag = "Default"; } ) .\AddRestrictedIPAzureAppService.ps1 ` -ResourceGroupName "YourResourceGroup" ` -AppServiceName "YourAppServiceName" ` -SubscriptionId "YourSubscriptionGuid" ` -NewIpRules $restrictions
- You need to have the Az PowerShell module installed.
After you run this script, the IP ranges you passed in will be added to the Access Restriction of your Azure App Service.
If you use Cloudflare or Availability Tests within Azure Application Insights, you'll need to include all of their IP's in order for those services to function.
The following two posts include scripts to grab and generate the IP Restrictions:
- Bulk add Cloudflare's IP addresses to Azure App Service Access Restrictions using Az PowerShell
- Bulk add Application Insights Availability Test IP addresses to Azure App Service Access Restrictions using Az PowerShell
Side note: The script is using hashtables because PSCustomObject is being parsed incorrectly by the Az module. See this GitHub issue for more information.