Changing Serilog Minimum level without application restart on .NET Framework and Core
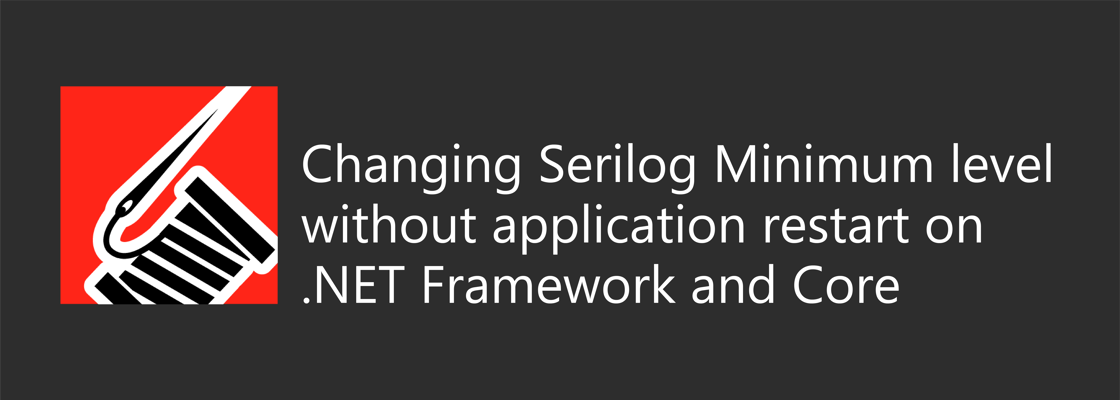
There are many ways to configure Serilog. The main way is to configure it using the fluent API in code, but you can also configure it using the classic .NET Framework AppSettings and the .NET Core Configuration library. The configuration library has the additional advantage that it supports dynamic reloading of the MinimumLevel
and LevelSwitches.
This means you can change the log level without reloading your application which is the closest you'll get to the `watch` feature in Log4Net.
Even though the Microsoft.Extensions.Configuration
libraries were introduced as part of .NET Core, these libraries are actually supported on .NET Framework as well. The Serilog documentation suggests that the Serilog.Settings.Configuration
package is for .NET Core, but you can also use it for .NET Framework!
Sample #
To configure Serilog using JSON Configuration with live reload on both .NET Framework and .NET Core, follow these steps:
- Create a console application using the .NET CLI/Visual Studio.
- Add the stable version of the following packages:
- Create a JSON file dedicated to Serilog configuration named
serilog.json
. On .NET Core, you can merge the configuration withappsettings.json
.
Add the following contents to the file:
{ "Serilog": { "LevelSwitches": { "$consoleSwitch": "Verbose", "$fileSwitch": "Verbose" }, "Using": [ "Serilog.Sinks.Console", "Serilog.Sinks.File" ], "MinimumLevel": "Verbose", "WriteTo": [ { "Name": "Console", "Args": { "levelSwitch": "$consoleSwitch" } }, { "Name": "File", "Args": { "path": "Logs/log.txt", "levelSwitch": "$fileSwitch" } } ] } }
- Make sure the
serilog.json
file will be copied alongside the output by updating the csproj-file:
<ItemGroup> <None Include="serilog.json"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> </ItemGroup>
You can also do this in Visual Studio by right-clicking the file, selecting 'properties' in the context menu, and then update the 'Copy to Output Directory' setting to 'Copy if Newer'.
- Update the
Program.cs
file to load theserilog.json
configuration and pass it to the Serilog builder:
using System; using System.IO; using System.Threading.Tasks; using Microsoft.Extensions.Configuration; using Serilog; class Program { static async Task Main(string[] args) { // load serilog.json to IConfiguration var configuration = new ConfigurationBuilder() .SetBasePath(Directory.GetCurrentDirectory()) // reloadOnChange will allow you to auto reload the minimum level and level switches .AddJsonFile(path: "serilog.json", optional: false, reloadOnChange: true) .Build(); // build Serilog logger from IConfiguration var log = new LoggerConfiguration() .ReadFrom.Configuration(configuration) .CreateLogger(); while (true) { log.Verbose("This is a Verbose log message"); log.Debug("This is a Debug log message"); log.Information("This is an Information log message"); log.Warning("This is a Warning log message"); log.Error("This is a Error log message"); log.Fatal("This is a Fatal log message"); await Task.Delay(1000); } } }
- Build your project and run it.
Serilog should output all log messages to the console and file every second. Do the following to try out the auto reloading:
- Modify the
MinimumLevel
property from "Verbose" to "Fatal" and watch how only Fatal messages are logged to the console and file atLogs/log.txt
. Revert this change. - Modify the
$consoleSwitch
from "Verbose" to "Fatal" and watch how only Fatal messages are logged to the console. All messages are still being logged to the file.
UsingLevelSwitches
you can control theMinimumLevel
of individual sinks such as console and file.
This means you could have a more verbose log level for a file and a less verbose level for Azure Application Insights to reduce costs.
To learn more about Serilog, check out the Serilog website.
Summary #
This tutorial demonstrates how you can configure Serilog using JSON Configuration on .NET Framework and .NET Core, including the auto reload feature for the minimum log level and level switches.