Using ConfigurationProviders from Microsoft.Extensions.Configuration on .NET Framework
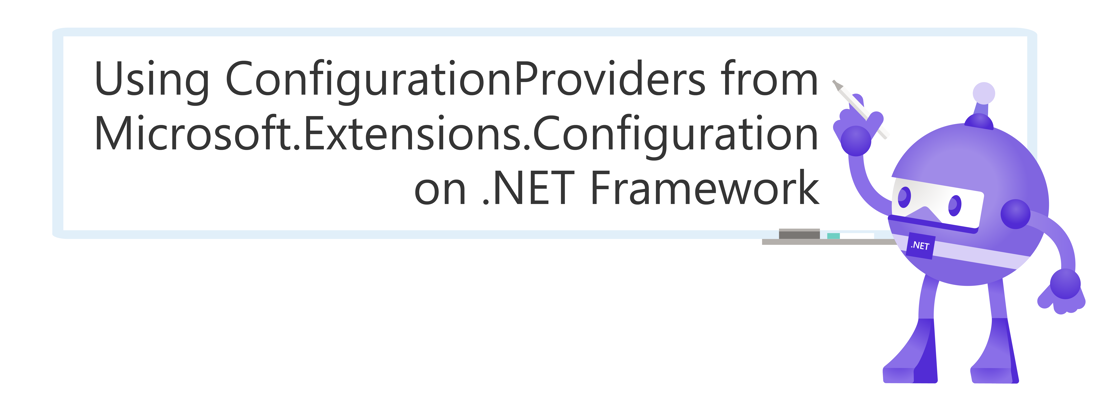
.NET Core changed a lot from .NET Framework and introduced many new libraries/API's. Some of those libraries are actually built to support multiple .NET platforms including .NET Framework. So, if you're still using .NET Framework, you could also take advantage of these new libraries.
Take the dotnet/extensions for example:
.NET Extensions is an open-source, cross-platform set of APIs for commonly used programming patterns and utilities, such as dependency injection, logging, and app configuration. Most of the API in this project is meant to work on many .NET platforms, such as .NET Core, .NET Framework, Xamarin, and others. While commonly used in ASP.NET Core applications, these APIs are not coupled to the ASP.NET Core application model. They can be used in console apps, WinForms and WPF, and others.
Source: dotnet/extensions repository
These libraries are very useful, but on top of that, many other open-source projects consume these same libraries. For example, Serilog is an open-source logging library and one way to configure Serilog is using the Microsoft.Extensions.Configuration library. When using this configuration library, you can change the log level of the logger without restarting your application because of the live reload capabilities in Microsoft.Extensions.Configuration. This is not possible out of the box with the normal way of configuring Serilog on .NET Framework.
Sample #
Follow these steps if you want to take the Microsoft.Extensions.Configuration libraries for a spin:
- Create a .NET Framework Console App using Visual Studio
- Add the following packages to your project using the NuGet package manager console:
Install-Package Microsoft.Extensions.Configuration.Json -Version 3.1.8 Install-Package Microsoft.Extensions.Configuration.CommandLine -Version 3.1.8 Install-Package Microsoft.Extensions.Configuration.Binder -Version 3.1.8
The Json package adds support for JSON files as configuration; the CommandLine package will help you convert the string[] args into configuration; the Binder package will bind configuration to typed objects.
- Create a json file at the root of your project named myconfig.json and add the following contents:
{ "name": "Best pizza", "toppings": [ "Marinara sauce", "Cheese", "More cheese", "Pineapple", "More pineapple" ] }
- Update the Program.cs file:
using Microsoft.Extensions.Configuration; using System; using System.Collections.Generic; using System.IO; class Program { static void Main(string[] args) { var configuration = new ConfigurationBuilder() .SetBasePath(Directory.GetCurrentDirectory()) // load in the json file as configuration .AddJsonFile(path: "myconfig.json", optional: false, reloadOnChange: true) // override configuration from the json file with commandline arguments .AddCommandLine(args) .Build(); } }
Now you can read out the data from the JSON file and the command line arguments using the configuration object.
- Instead of manually extracting the configuration, you can use the Bind function to bind the configuration data to a typed object. Update the Program.cs file with the binding code:
using Microsoft.Extensions.Configuration; using System; using System.Collections.Generic; using System.IO; class Program { static void Main(string[] args) { var configuration = new ConfigurationBuilder() .SetBasePath(Directory.GetCurrentDirectory()) // load in the json file as configuration .AddJsonFile(path: "myconfig.json", optional: false, reloadOnChange: true) // override configuration from the json file with commandline arguments .AddCommandLine(args) .Build(); var pizza = new Pizza(); configuration.Bind(pizza); Console.WriteLine($"Pizza name: {pizza.Name}"); Console.WriteLine($"Pizza toppings:"); foreach (var topping in pizza.Toppings) { Console.WriteLine($"- {topping}"); } Console.WriteLine("Press any enter to exit"); Console.ReadLine(); } } public class Pizza { public string Name { get; set; } public List<string> Toppings { get; set; } }
To bind the configuration, you need to first create a new typed class, create a new instance of that class, and then pass it to configuration.Bind.
The output of this little program will look like this:
Pizza name: Best pizza Pizza toppings: - Marinara sauce - Cheese - More cheese - Pineapple - More pineapple Press any enter to exit
You can modify the configuration by passing command line arguments:
./ConfigurationBuilderOnFramework.exe /toppings:4 "Mushrooms" /toppings:5 "Sausage" # Output: # Pizza name: Best pizza # Pizza toppings: # - Marinara sauce # - Cheese # - More cheese # - Pineapple # - Mushrooms # - Sausage # Press any enter to exit
In addition to the JSON and Command Line provider, you can take advantage of many more providers. Search for "Microsoft.Extensions.Configuration" at NuGet.org and you will find many more such as:
- Microsoft.Extensions.Configuration.EnvironmentVariables
- Microsoft.Extensions.Configuration.UserSecrets
- Microsoft.Extensions.Configuration.Ini
- Microsoft.Extensions.Configuration.Xml
- Microsoft.Extensions.Configuration.AzureKeyVault
- Microsoft.Extensions.Configuration.KeyPerFile
- Microsoft.Extensions.Configuration.AzureAppConfiguration
Summary #
Even though many of the new API's/libraries/packages are introduced with .NET Core, some of them are also compatible with other .NET Platforms such as .NET Framework, Xamarin, etc. The new configuration API's are very powerful and can be used on .NET Framework.